Python Ariadne, how to change status code from 400 to 200.
Vue Apollo client throws an error when receives a HTTP response whose status code is 400.
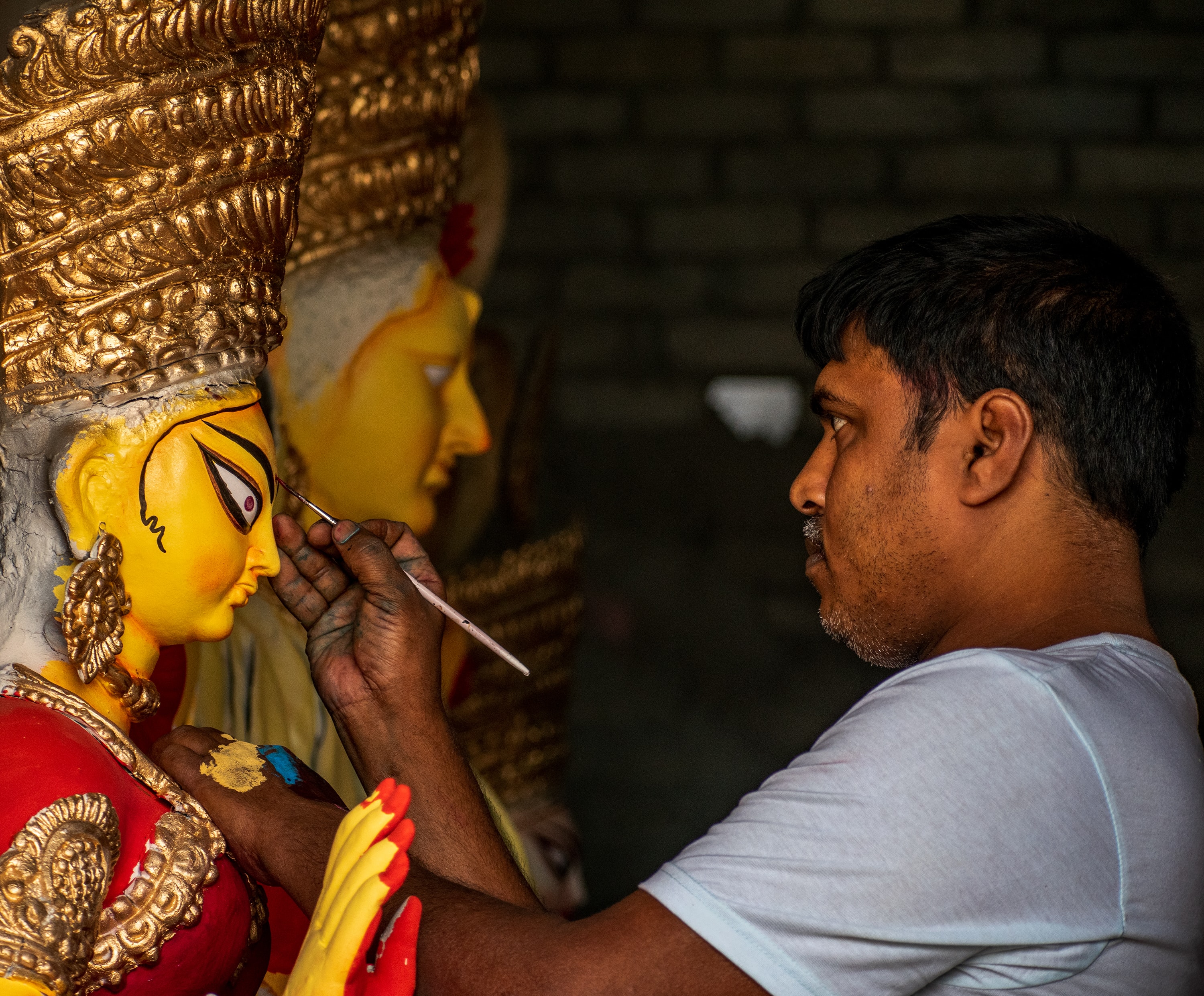
"Ariadne" is Python's GraphQL web framework. I think this framework is so nice. And "Apollo client" is most popular GraphQL client. When Ariadne return an error, this framework return a response with HTTP STATUS CODE 400. HTTP SATUS CODE 400 makes "Apollo client" throw an error.
Then "Apollo client" is unable to properly handle the error from Ariadne,
because we cannot get a result.errors
properly.
So even nasty solution, I create a middleware which force HTTP STATUS CODE change from 400 to 200.
#
# usage
#
app = middleware(
app
)
#
# definition
#
from starlette.types import ASGIApp, Message, Receive, Scope, Send
def middleware(
internal_app: ASGIApp
):
"""Change HTTP STATUS CODE from 400 to 200."""
async def outer_app(
scope: Scope,
receive: Receive,
send: Send
):
async def send_wrapper(
message: Message
):
if 'status' in message and message['status'] == 400:
message['status'] = 200
await send(message)
await internal_app(scope, receive, send_wrapper)
return outer_app